剑指 Offer 35. 复杂链表的复制
1. Question
请实现 copyRandomList
函数,复制一个复杂链表。在复杂链表中,每个节点除了有一个 next
指针指向下一个节点,还有一个 random
指针指向链表中的任意节点或者 null
。
2. Examples
示例 1:
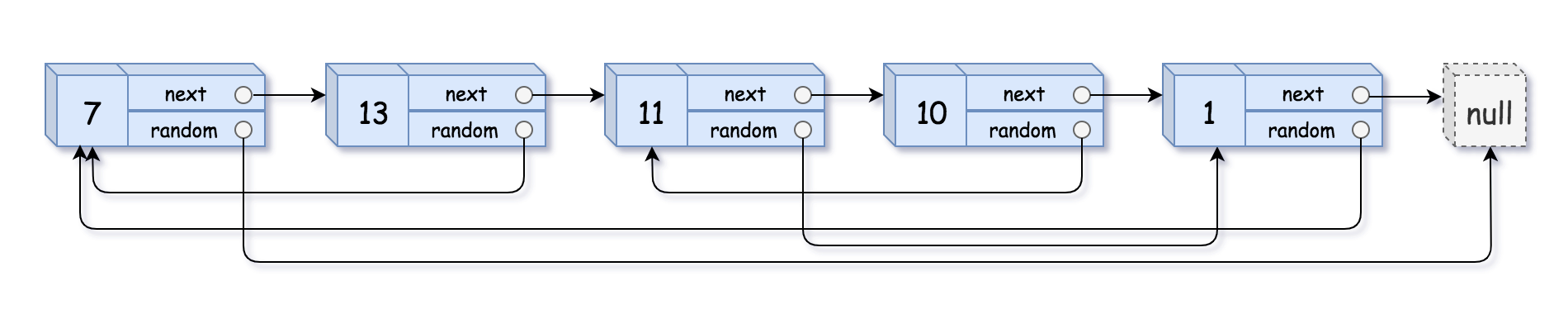
输入:head = [[7,null],[13,0],[11,4],[10,2],[1,0]]
输出:[[7,null],[13,0],[11,4],[10,2],[1,0]]
示例 2:

输入:head = [[1,1],[2,1]]
输出:[[1,1],[2,1]]
示例 3:

输入:head = [[3,null],[3,0],[3,null]]
输出:[[3,null],[3,0],[3,null]]
示例 4:
输入:head = []
输出:[]
解释:给定的链表为空(空指针),因此返回 null。
3. Constraints
-10000 <= Node.val <= 10000
Node.random
为空(null)或指向链表中的节点。- 节点数目不超过 1000 。
4. References
来源:力扣(LeetCode) 链接:https://leetcode-cn.com/problems/fu-za-lian-biao-de-fu-zhi-lcof 著作权归领扣网络所有。商业转载请联系官方授权,非商业转载请注明出处。
5. Solutions
通过HashMap映射新旧的Node。
/*
// Definition for a Node.
class Node {
int val;
Node next;
Node random;
public Node(int val) {
this.val = val;
this.next = null;
this.random = null;
}
}
*/
class Solution {
public Node copyRandomList(Node head) {
if (head == null) {
return null;
}
Node oldHead = head;
Node newHead = new Node(oldHead.val);
Node ans = newHead;
HashMap<Node, Node> map = new HashMap<>();
map.put(oldHead, newHead);
while(oldHead.next != null) {
Node node = new Node(oldHead.next.val);
newHead.next = node;
oldHead = oldHead.next;
newHead = newHead.next;
map.put(oldHead, newHead);
}
newHead = ans;
oldHead = head;
while(oldHead != null) {
newHead.random = map.get(oldHead.random);
newHead = newHead.next;
oldHead = oldHead.next;
}
return ans;
}
}